How to add google address auto complete with map in angular 2023
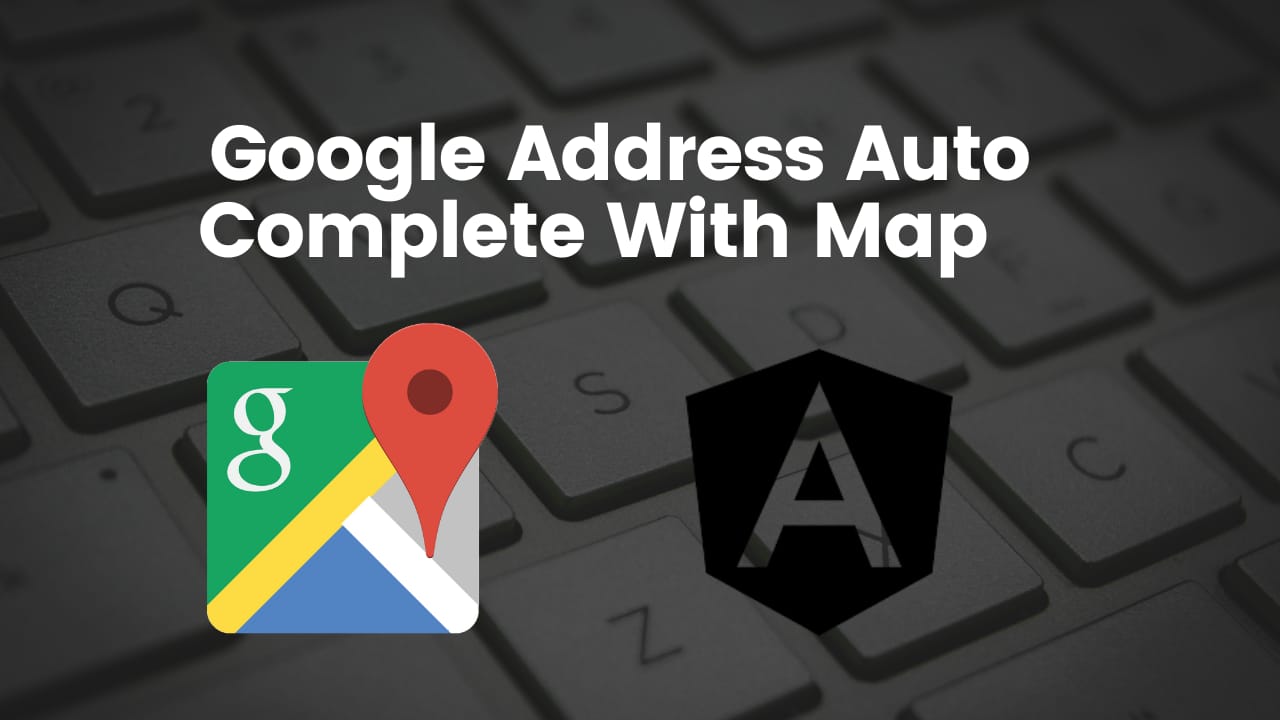
In this article I’m going to show you how you can implement Google Autocomplete with Map in angular.
Google Maps is a powerful tool that allows developers to add maps, geolocation, and location-based services to their web and mobile applications. One of the most useful features of Google Maps is the ability to add an address autocomplete input, which can help users search for and select a specific location more easily.
Prerequisites
Before we get started, you will need the following:
- An Angular application. If you don't have one, you can create a new Angular project by running the following command: ng new project-name.
- A Google Maps API key. You can obtain an API key by following the instructions here.
- ngx-google-places-autocomplete NPM Package
- @agm/core NPM Package
Step 1 – Create New Angular Project
Create a new project by running following command in terminal
ng new googleaddress-autocomplete
Step 2 – Install ngx-google-places-autocomplete NPM Package
Install ngx-google-places-autocomplete by running following command in terminal
npm install ngx-google-places-autocomplete
Step 3 – Install the Angular Google Maps NPM Package
Install ngx-google-places-autocomplete by running following command in terminal
npm install @agm/core@1.1.0 –save
Note:- Latest version of @agm/core has a few bugs also it's in the beta state. That’s why I’m using version 1.1.0
Step 4 – Import Modules in app.module.ts File
After installing all the above packages go the the module file where your component registered.
In my case I don’t have any other modules so I imported in app.module.ts file.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
//import for GooglePlaceModule
import { GooglePlaceModule } from "ngx-google-places-autocomplete";
import { AgmCoreModule } from '@agm/core'; // import this for show address on map
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
GooglePlaceModule,
AgmCoreModule.forRoot({
apiKey: 'YOUR_API_KEY'
})
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
After importing all package add google map script In your index.html file
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>GoogleaddressAutocomplete</title>
<base href="/" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link rel="icon" type="image/x-icon" href="favicon.ico" />
<!-- Google Map Script -->
<script
async
src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY_HERE&libraries=places"
></script>
<!-- Replace YOUR_API_KEY_HERE with your google API Key-->
</head>
<body>
<app-root></app-root>
</body>
</html>
Step 5 – Adding Autocomplete and showing on map functionality in our component
in this step we are going to create HTML view first.
paste the below code in your app.component.html
<div class="container">
<label for="search">Search Address: </label>
<!-- If you want to remove country restriction then remove options property from below -->
<input
[options]="options"
type="search"
ngx-google-places-autocomplete
(onAddressChange)="handleAddressChanges($event)"
/>
<br />
<br />
<p>Address: {{ address }}</p>
<p>Latitude: {{ lat }}</p>
<p>Longitude: {{ long }}</p>
<br />
<br />
<ng-container *ngIf="lat !== undefined && long !== undefined">
<agm-map [latitude]="lat" [longitude]="long" [zoom]="zoom">
<agm-marker
[latitude]="lat"
[longitude]="long"
[markerDraggable]="true"
(dragEnd)="markerDragEnd($event)"
></agm-marker>
</agm-map>
</ng-container>
</div>
Open app.component.css file and paste below code
agm-map{
height: 200px;
width: 500px;
}
After Complete HTML and CSS part open app.component.ts file and paste below code
import { Component } from '@angular/core';
import { MapsAPILoader } from "@agm/core";
declare var google: any;
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'googleaddress-autocomplete';
address: any;
lat: any;
long: any;
zoom: number = 16;
private geoCoder = new google.maps.Geocoder();
// if you want to restrict the country
options: any = {
componentRestrictions: {
country: ["IN"],
},
};
constructor(private mapsAPILoader: MapsAPILoader) { }
handleAddressChanges(address: any) {
this.address = address.formatted_address
this.lat = address.geometry.location.lat()
this.long = address.geometry.location.lng()
}
// drag marker to change the location
markerDragEnd($event: any) {
this.lat = $event.coords.lat;
this.long = $event.coords.lng;
this.getAddress($event.coords.lat, $event.coords.lng);
}
getAddress(latitude: any, longitude: any) {
this.geoCoder.geocode(
{ location: { lat: latitude, lng: longitude } },
(results: any, status: any) => {
if (status === "OK") {
if (results[0]) {
this.zoom = 16;
this.lat = latitude
this.long = longitude
this.address = results[0].formatted_address;
} else {
window.alert("No results found");
}
} else {
window.alert("Geocoder failed due to: " + status);
}
}
);
}
}
Step 6: Test the Google Address Autocomplete With Map
Now that we have everything set up, let's test the autocomplete input. Run your Angular application by running ng serve –o command and try typing in an address into the input field. You should see a dropdown list of suggestions appear as you type. When you select a suggestion from the list, the map should update to show the location of the selected address.
If you drag the marker then it will show the marker position address.
That's it! You now have a working Google Maps address autocomplete with Map
Conclusion
In this tutorial, we learned how to add a Google Maps address autocomplete with a map to an Angular application using the Angular Google Maps (AGM) library. We also learned how to change the address by dragging the marker on the map.
I hope this tutorial was helpful, and if you have any questions or comments, please let me know in the comments section below.
Leave a comment